Xamarin.Forms and Realm: CRUD Example
Monday, August 07, 2017
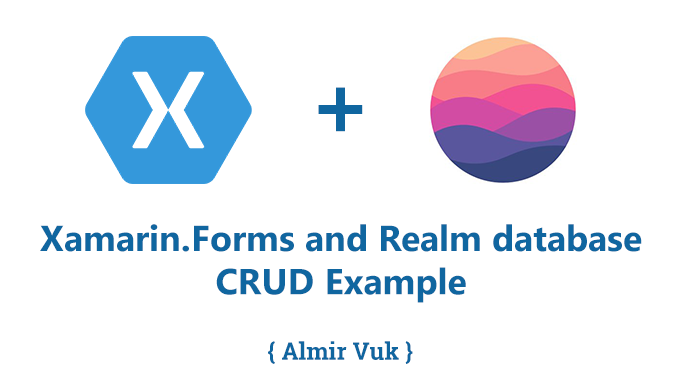
For all of you who are not familiar with Realm here is a few sentences about it from their web site. Note: I will make another blog post about pros and cons, in the next blog posts.
"The Realm Mobile Database is built from the ground up to run on mobile devices. Unlike a traditional database, objects in a Realm are native objects. You don’t have to copy objects out of the database, modify them, and save them back—you’re always working with the “live,” real object. If one thread or process modifies an object, other threads and processes can be immediately notified. Objects always stay in sync.
The Realm Mobile Database is open source (using the Apache license) and cross-platform, with libraries available for Android, iOS, Xamarin (.NET), and React Native. Realms are fully interchangeable between platforms.
You can use the Realm Mobile Database all on its own." - From https://realm.io/docs/get-started/overview/
I was searching for some SQLite alternatives and when I first saw Realm I was:
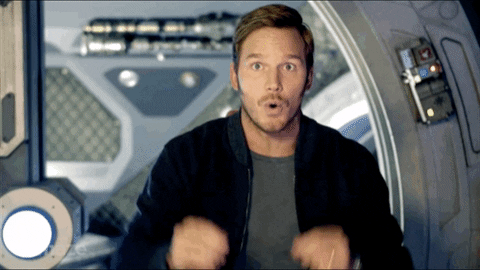
It is very easy to begin with it, couple of nuget packages and you are ready to code a model and data access layer for your mobile app.
This tutorial will show you how to achieve CRUD in your app using Realm as mobile database.
So let's begin.
SETUP:
Create new Xamarin.Forms app, in my example I will be using PCL for "shared" code project.As always add three standard folders: Models, Views and ViewModels, we will use MVVM for our project as architectural pattern.
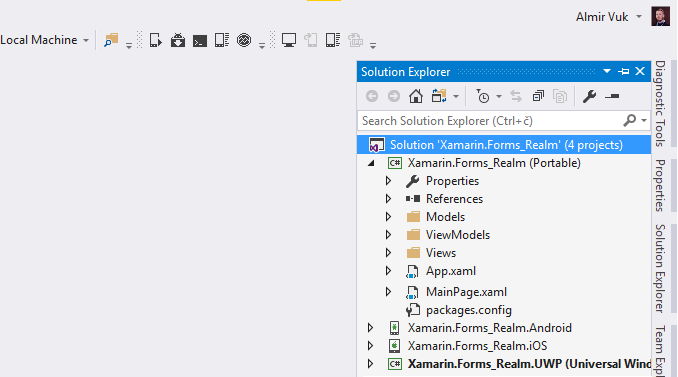
In order to use Realm database we need to add nuget package for our projects, so go to the Manage Nuget Packages for solution, and find Realm nuget like this:
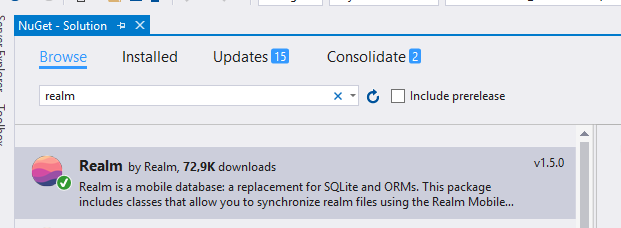
Make sure that you install it on all projects: PCL and others (Android, UWP, iOS), wait couple of second and it will install some other nugets that are depending on this main nuget.
... and you are good to go.
This app will be about teams and players, so in the Models folder we will create two classes which will represent the Team and Player.
We have one-to-many relationship between team and player, so inside of Player as "foreign" property we will have property of type Team, on the other side at Team class we will have one List of type Player. Using setup like this we have our one-to-many relationship between these two classes.
To start using Realm as mobile database, we need to "create tables" in database, in order to do that classes which will be tables need to inherent from RealmObject.
Our two classes looks like this:
Team.cs:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System; | |
using System.Collections.Generic; | |
namespace Xamarin.Forms_Realm.Models { | |
public class Team : RealmObject { | |
[PrimaryKey] | |
public string TeamId { get; set; } = Guid.NewGuid().ToString(); | |
public string Title { get; set; } | |
public string Manager { get; set; } | |
public string City { get; set; } | |
public string StadiumName { get; set; } | |
public IList<Player> Players { get; } | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System; | |
namespace Xamarin.Forms_Realm.Models { | |
public class Player : RealmObject{ | |
[PrimaryKey] | |
public string PlayerId { get; set; } = Guid.NewGuid().ToString(); | |
public string Name { get; set; } | |
public int JerseyNumber { get; set; } | |
public string Position { get; set; } | |
public Team Team { get; set; } | |
} | |
} |
As you can see to declare primary key I am using data annotation [PrimaryKey] for property of the type string and it is autogenerated Guid, it is important to note that auto increment primary key is not supported yet in Realm.
Now we are ready we have our models with relationships, we need to start querying the data.
READ:
Now create one View/Page in our Views folder callled TeamsListPage.xaml and it looks like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8" ?> | |
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms" | |
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" | |
x:Class="Xamarin.Forms_Realm.Views.TeamsListPage" | |
Title="All Teams"> | |
<ContentPage.ToolbarItems> | |
<ToolbarItem Text="Add new" | |
Icon="ic_add.png" | |
Command="{Binding AddTeamCommand}" /> | |
</ContentPage.ToolbarItems> | |
<ContentPage.Content> | |
<ListView ItemsSource="{Binding AllTeams}" | |
HasUnevenRows="True" | |
ItemTapped="TeamTapped" | |
BackgroundColor="#f5f5f5"> | |
<ListView.ItemTemplate> | |
<DataTemplate> | |
<ViewCell> | |
<Grid BackgroundColor="White" | |
Margin="4"> | |
<Grid.RowDefinitions> | |
<RowDefinition Height="Auto" /> | |
<RowDefinition Height="Auto" /> | |
<RowDefinition Height="Auto" /> | |
</Grid.RowDefinitions> | |
<Label Grid.Row="0" | |
Text="{Binding Title}" | |
FontSize="Medium" | |
Margin="4" | |
FontAttributes="Bold" /> | |
<StackLayout Orientation="Horizontal" | |
Grid.Row="1" | |
Margin="4" | |
Padding="2"> | |
<Label Text="{Binding StadiumName}" | |
FontSize="Small" /> | |
<Label Text="{Binding City}" | |
FontSize="Small" /> | |
</StackLayout> | |
</Grid> | |
</ViewCell> | |
</DataTemplate> | |
</ListView.ItemTemplate> | |
</ListView> | |
</ContentPage.Content> | |
</ContentPage> |
At the top we have one toolbar item for action of opening new page and adding new teams in the database.
Main part of our view is a ListView control, item source of this ListView is binding to the List in our view-model that we will create after this step.
In side of ItemTemplate we are making the structure of ListView items (how will ListView item will look like), and Labels inside of grid are binding to the properties of list items of type Team, because this ListView will be for listing the Teams in our database.
Before creating a view-models I will add one BaseViewModel class in folder ViewModels, basically this class will hold the OnPropertyChanged method, and every view-model will inherit from it and we will be able to call OnPropertyChanged method for our properties.
BaseViewModel looks like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.Collections.Generic; | |
using System.ComponentModel; | |
using System.Linq; | |
using System.Runtime.CompilerServices; | |
using System.Text; | |
using System.Threading.Tasks; | |
using Xamarin.Forms; | |
namespace Xamarin.Forms_Realm.ViewModels { | |
public class BaseViewModel : INotifyPropertyChanged { | |
public event PropertyChangedEventHandler PropertyChanged; | |
protected void OnPropertyChanged(string propertyName = "") => | |
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); | |
} | |
} |
Now when we have our view, we can create our view model, in folder ViewModels add new class called TeamListViewModel.cs:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System; | |
using System.Collections.Generic; | |
using System.Collections.ObjectModel; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using System.Windows.Input; | |
using Xamarin.Forms; | |
using Xamarin.Forms_Realm.Models; | |
using Xamarin.Forms_Realm.Views; | |
namespace Xamarin.Forms_Realm.ViewModels { | |
public class TeamListViewModel : BaseViewModel{ | |
private ObservableCollection<Team> allTeams; | |
public ObservableCollection<Team> AllTeams { | |
get { return allTeams; } | |
set { allTeams = value; | |
} | |
} | |
public ICommand AddTeamCommand { get; private set; } | |
public TeamListViewModel() { | |
Realm context = Realm.GetInstance(); | |
AllTeams = new ObservableCollection<Team>(context.All<Team>()); | |
AddTeamCommand = new Command(async ()=> await Application.Current.MainPage.Navigation.PushAsync(new AddTeamPage())); | |
} | |
} | |
} |
At line 28: We are creating new object of type Realm and that object is basically representing our mobile database.
Using it we can do CRUD operations with it.
A line 30: I am initializing our ObservableCollection called AllTeams with result from context.All
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
context.All<TYPE_OF_CLASS_TO_GET>(): |
As you can see querying the data is very similar, not same but similar to EF style, later in this course we will do "Where" and other condition on lists.
And at the end of the constructor we are initializing the AddTeamCommand and when users click on our toolbar item we will navigate to AddTeamPage which we will create in next steps.
Also do not forget to set binding context to the ViewModel from your view code-behind.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using Xamarin.Forms; | |
using Xamarin.Forms.Xaml; | |
using Xamarin.Forms_Realm.Models; | |
using Xamarin.Forms_Realm.ViewModels; | |
namespace Xamarin.Forms_Realm.Views { | |
[XamlCompilation(XamlCompilationOptions.Compile)] | |
public partial class TeamsListPage : ContentPage { | |
public TeamsListPage() { | |
InitializeComponent(); | |
} | |
protected override void OnAppearing() { | |
BindingContext = new TeamListViewModel(); | |
} | |
private async void TeamTapped(object sender, ItemTappedEventArgs e) { | |
// TODO | |
} | |
} | |
} |
Now when we have our page for listing the teams, we need to make a view for adding (Creating) new teams.
CREATE:
In the Views folder create new page called AddTeamPage.xaml, and it should look like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8" ?> | |
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms" | |
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" | |
x:Class="Xamarin.Forms_Realm.Views.AddTeamPage" | |
Title="Add new team"> | |
<ContentPage.ToolbarItems> | |
<ToolbarItem Text="Save" | |
Icon="ic_save.png" | |
Command="{Binding SaveTeamCommand}" /> | |
</ContentPage.ToolbarItems> | |
<ContentPage.Content> | |
<StackLayout> | |
<Entry Placeholder="Team Name/Title" | |
Text="{Binding Title}" | |
Margin="4" /> | |
<Entry Placeholder="Manager name" | |
Text="{Binding Manager}" | |
Margin="4" /> | |
<Entry Placeholder="City name" | |
Text="{Binding City}" | |
Margin="4" /> | |
<Entry Placeholder="Name of the stadium" | |
Text="{Binding StadiumName}" | |
Margin="4" /> | |
</StackLayout> | |
</ContentPage.Content> | |
</ContentPage> |
As you can see it is very simple "input form" page with couple of Entry controls for entering data for Team properties, and one toolbar item which handle the save/create operation on the database.
Next step as always is to add view-model and following our convention it will be AddTeamViewModel.cs:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System.Windows.Input; | |
using Xamarin.Forms; | |
using Xamarin.Forms_Realm.Models; | |
namespace Xamarin.Forms_Realm.ViewModels { | |
public class AddTeamViewModel : BaseViewModel{ | |
private string title; | |
public string Title { | |
get { return title; } | |
set { title = value; | |
OnPropertyChanged("Title"); | |
} | |
} | |
private string manager; | |
public string Manager { | |
get { return manager; } | |
set { manager = value; | |
OnPropertyChanged("Manager"); | |
} | |
} | |
private string city; | |
public string City { | |
get { return city; } | |
set { city = value; | |
OnPropertyChanged("City"); | |
} | |
} | |
private string stadiumName; | |
public string StadiumName { | |
get { return stadiumName; } | |
set { stadiumName = value; | |
OnPropertyChanged("StadiumName"); | |
} | |
} | |
public ICommand SaveTeamCommand { get; private set; } | |
public AddTeamViewModel() { | |
SaveTeamCommand = new Command(SaveTeam); | |
} | |
async void SaveTeam() { | |
Realm context = Realm.GetInstance(); | |
context.Write(() => { | |
context.Add<Team>(new Team() { City = City, StadiumName = StadiumName, Title = Title, Manager = Manager }); | |
}); | |
/* | |
* Also you can use it like this | |
* using (var transaction = context.BeginWrite()) { | |
* context.Add<Team>(new Team() { City = City, StadiumName = StadiumName, Title = Title, Manager = Manager }); | |
* transaction.Commit(); | |
* } | |
*/ | |
// After adding new entry to database close this page | |
await Application.Current.MainPage.Navigation.PopAsync(); | |
} | |
} | |
} |
Our view-model is very simple we have couple of properties, inside of the setter you can see OnPropertyChanged method, standard MVVM approach, when property is changed controls from XAML which are binding to it will have updated value.
On my blog I have couple of MVVM blog posts so you can check it if this is new to you.
Also we have one Command which will invoke method SaveTeam() where is located the main part of our view-model.
Inside of SaveTeam we have again Realm object named context. Below it there is a two ways of making insert in the the db.
You can use context.Write() and inside of the new delegate there is a main part of inserting like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
context.Add<TYPE_OF_OBJECT_TO_INSERT>(OBJECT_TO_INSERT); |
... and after adding new entry to database close this page.
Do not forget to add binding context to code behind of our view.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Xamarin.Forms; | |
using Xamarin.Forms.Xaml; | |
using Xamarin.Forms_Realm.ViewModels; | |
namespace Xamarin.Forms_Realm.Views { | |
[XamlCompilation(XamlCompilationOptions.Compile)] | |
public partial class AddTeamPage : ContentPage { | |
public AddTeamPage() { | |
InitializeComponent(); | |
} | |
protected override void OnAppearing() { | |
BindingContext = new AddTeamViewModel(); | |
base.OnAppearing(); | |
} | |
} | |
} |
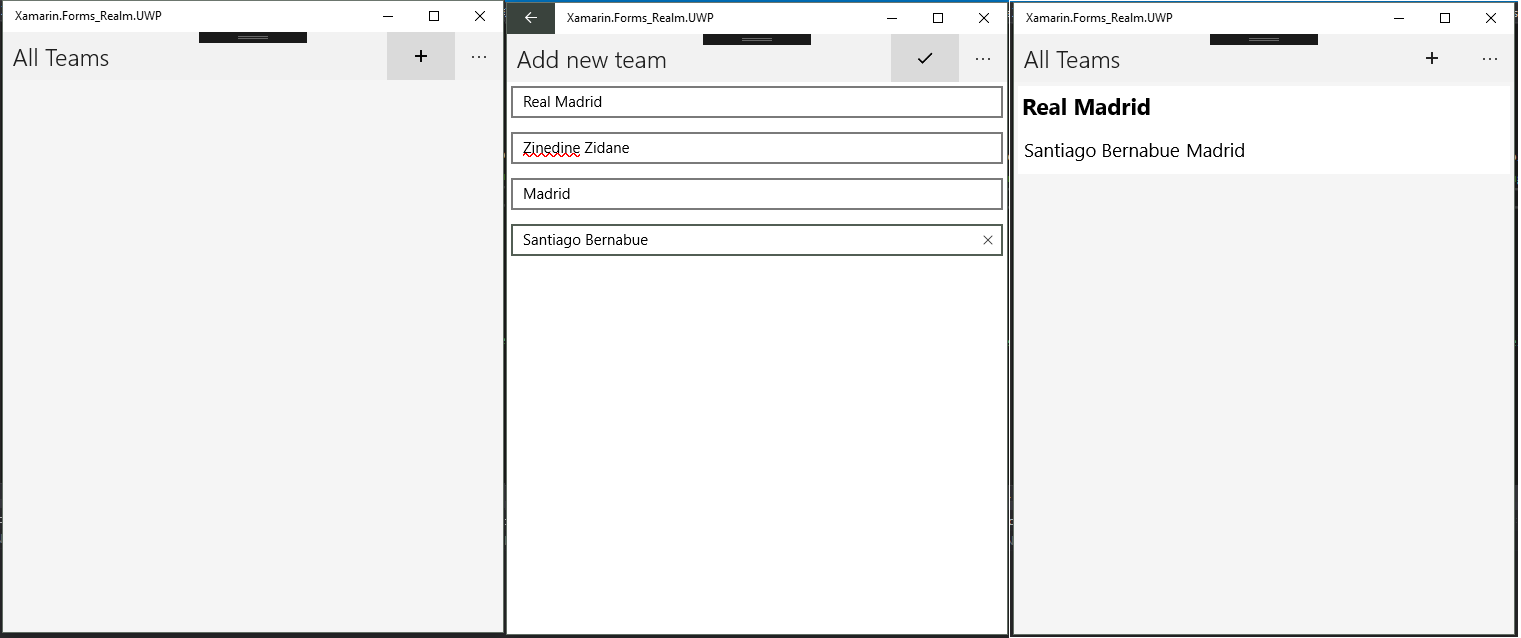
It works! 😀
So we can continue with tutorial.
DELETE:
Next step is to show details about team and add players to it, and also delete the team if we want.First step is to create TeamDetailsPage.xaml in our views folder.
Like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8" ?> | |
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms" | |
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" | |
x:Class="Xamarin.Forms_Realm.Views.TeamDetailsPage" | |
Title="About team"> | |
<ContentPage.ToolbarItems> | |
<ToolbarItem Text="Add player" | |
Command="{Binding AddPlayerCommand}" | |
Icon="ic_add_player.png" /> | |
<ToolbarItem Text="Edit team" | |
Icon="ic_edit.png" | |
Command="{Binding EditTeamCommand}" /> | |
<ToolbarItem Text="Delete team" | |
Icon="ic_delete.png" | |
Command="{Binding DeleteTeamCommand}" /> | |
</ContentPage.ToolbarItems> | |
<ContentPage.Content> | |
<ScrollView> | |
<Grid> | |
<Grid.RowDefinitions> | |
<RowDefinition Height="Auto" /> | |
<RowDefinition Height="Auto" /> | |
<RowDefinition Height="Auto" /> | |
<RowDefinition Height="Auto" /> | |
<RowDefinition Height="Auto"/> | |
</Grid.RowDefinitions> | |
<Label Text="{Binding Title}" | |
Margin="8" | |
FontSize="Medium" | |
Grid.Row="0" /> | |
<Label Text="{Binding Manager}" | |
Grid.Row="1" | |
FontSize="Small" | |
Margin="8" /> | |
<Label Text="{Binding City}" | |
Grid.Row="2" | |
FontSize="Small" | |
Margin="8" /> | |
<Label Text="{Binding StadiumName}" | |
Grid.Row="3" | |
FontSize="Small" | |
Margin="8" /> | |
<StackLayout Grid.Row="4" | |
Margin="8"> | |
<Label Text="Players:" | |
FontSize="Small" | |
Margin="4" /> | |
<ListView ItemsSource="{Binding Players}" | |
HasUnevenRows="True" | |
ItemTapped="OnPlayerTapped" | |
Margin="4"> | |
<ListView.ItemTemplate> | |
<DataTemplate> | |
<ViewCell> | |
<StackLayout Orientation="Horizontal" | |
HorizontalOptions="StartAndExpand"> | |
<Label Text="{Binding Name}" | |
FontSize="Small" | |
Margin="4"/> | |
<Label Text="{Binding Position}" | |
FontSize="Small" | |
Margin="4" /> | |
<Label Text="{Binding JerseyNumber}" | |
FontSize="Small" | |
Margin="4"/> | |
<Image Source="ic_edit.png" | |
HeightRequest="17" | |
HorizontalOptions="End" | |
VerticalOptions="Center" | |
Margin="4" /> | |
</StackLayout> | |
</ViewCell> | |
</DataTemplate> | |
</ListView.ItemTemplate> | |
</ListView> | |
</StackLayout> | |
</Grid> | |
</ScrollView> | |
</ContentPage.Content> | |
</ContentPage> |
After that we have couple of labels which will hold the data for the Team, and below them there is a simple ListView for the players of that team.
Actions are: add new player, edit this team and delete it.
For now let's start with view-model for this details view:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System.Collections.ObjectModel; | |
using System.Linq; | |
using System.Windows.Input; | |
using Xamarin.Forms; | |
using Xamarin.Forms_Realm.Models; | |
using Xamarin.Forms_Realm.Views; | |
namespace Xamarin.Forms_Realm.ViewModels { | |
public class TeamDetailsViewModel : BaseViewModel { | |
private string title; | |
public string Title { | |
get { return title; } | |
set { | |
title = value; | |
OnPropertyChanged("Title"); | |
} | |
} | |
private string manager; | |
public string Manager { | |
get { return manager; } | |
set { | |
manager = value; | |
OnPropertyChanged("Manager"); | |
} | |
} | |
private string city; | |
public string City { | |
get { return city; } | |
set { | |
city = value; | |
OnPropertyChanged("City"); | |
} | |
} | |
private string stadiumName; | |
public string StadiumName { | |
get { return stadiumName; } | |
set { | |
stadiumName = value; | |
OnPropertyChanged("StadiumName"); | |
} | |
} | |
private ObservableCollection<Player> players; | |
public ObservableCollection<Player> Players { | |
get { return players; } | |
set { players = value; } | |
} | |
public ICommand AddPlayerCommand { get; private set; } | |
public ICommand EditTeamCommand { get; private set; } | |
public ICommand DeleteTeamCommand { get; private set; } | |
private string _teamId; | |
public TeamDetailsViewModel(string teamId) { | |
_teamId = teamId; | |
Realm context = Realm.GetInstance(); | |
var team = context.Find<Team>(teamId); | |
// Setting property values from team object | |
// that we get from database | |
Title = team.Title; | |
City = team.City; | |
StadiumName = team.StadiumName; | |
Manager = team.Manager; | |
// You can not do like this: | |
// Players = new ObservableCollection<Player>(context.All<Player>().Where(p => p.Team.TeamId == teamId)); | |
// Querying by nested RealmObjects attributes is not currently supported: | |
Players = new ObservableCollection<Player>(context.All<Player>().Where(p => p.Team == team)); | |
// Commands for toolbar items | |
AddPlayerCommand = new Command(async () => await Application.Current.MainPage.Navigation.PushAsync(new AddPlayerPage(team.TeamId))); | |
EditTeamCommand = new Command(async () => await Application.Current.MainPage.Navigation.PushAsync(new EditTeamPage(team.TeamId))); | |
DeleteTeamCommand = new Command(DeleteTeam); | |
} | |
void DeleteTeam() { | |
Realm context = Realm.GetInstance(); | |
var team = context.Find<Team>(_teamId); | |
context.Write(() => { | |
context.Remove(team); | |
}); | |
Application.Current.MainPage.Navigation.PopAsync(); | |
} | |
} | |
} |
First thing that this view-model does in the constructor we are passing the teamId which is passed from TeamsListPage.xaml.cs from event called TeamTapped so take a look at that implementation.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using Xamarin.Forms; | |
using Xamarin.Forms.Xaml; | |
using Xamarin.Forms_Realm.Models; | |
using Xamarin.Forms_Realm.ViewModels; | |
namespace Xamarin.Forms_Realm.Views { | |
[XamlCompilation(XamlCompilationOptions.Compile)] | |
public partial class TeamsListPage : ContentPage { | |
public TeamsListPage() { | |
InitializeComponent(); | |
} | |
protected override void OnAppearing() { | |
BindingContext = new TeamListViewModel(); | |
} | |
private async void TeamTapped(object sender, ItemTappedEventArgs e) { | |
if (e.Item != null) { | |
var team = (Team)e.Item; | |
await Navigation.PushAsync(new TeamDetailsPage(team.TeamId)); | |
} | |
} | |
} | |
} |
This is a common way to get value from tapped item in ListView and we are navigating to the TeamDetailsPage and passing the teamId value to it.
TeamDetailsPage.xaml.cs looks like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using Xamarin.Forms; | |
using Xamarin.Forms.Xaml; | |
using Xamarin.Forms_Realm.Models; | |
using Xamarin.Forms_Realm.ViewModels; | |
namespace Xamarin.Forms_Realm.Views { | |
[XamlCompilation(XamlCompilationOptions.Compile)] | |
public partial class TeamDetailsPage : ContentPage { | |
private string _teamId; | |
public TeamDetailsPage(string teamId) { | |
InitializeComponent(); | |
_teamId = teamId; | |
} | |
protected override void OnAppearing() { | |
BindingContext = new TeamDetailsViewModel(_teamId); | |
base.OnAppearing(); | |
} | |
private void OnPlayerTapped(object sender, ItemTappedEventArgs e) { | |
if (e.Item != null) { | |
var player = (Player)e.Item; | |
Navigation.PushAsync(new EditPlayerPage(player.PlayerId)); | |
} | |
} | |
} | |
} |
Also here we are implemented OnPlayerTapped event where we are opening the EditPlayerPage will we will cover in next steps, for now let't go back to our TeamDetailsViewModel.
After ObservableCollection there is a couple of command for toolbar items.
In the constructor using teamId we are setting it to the private field _teamId to use it outside of constructor.
Now again we are creating Realm object from GetInstance method.
and new object called team will be created from database, we are fetch it using generic method called Find with this syntax:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
context.Find<TYPE_OF_CLASS_TO_GET>(PRIMARY_KEY); |
We are initializing observable collection from database, and we are using ".All" method, ".All" method is returning the type IQueryable so we can use LINQ without any problems, here you can see that I use Where condition to get only those players which are playing for that team.
First time I was writing this, I was trying to set condition like this: .Where(p => p.Team.TeamId == _teamId)
but as you can see from my comments "Querying by nested RealmObjects attributes is not currently supported".
Bellow you can see couple of command initialization, last one for deleting object is one that I want to cover in this part of tutorial.
Again we create Realm context instance, find this team using Find method via teamId.
You need to open Write method and inside of it with very simple Remove method we can delete our object from database.
Remove method is accepting RealmObject as parameter and that is it our team will be deleted in this case.
You can test this part if you want (of course you want).
Next step is to see how to Edit/Update objects in Realm.
UPDATE:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8" ?> | |
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms" | |
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" | |
x:Class="Xamarin.Forms_Realm.Views.EditTeamPage" | |
Title="Edit team"> | |
<ContentPage.ToolbarItems> | |
<ToolbarItem Text="Save" | |
Icon="ic_save.png" | |
Command="{Binding SaveTeamCommand}" /> | |
</ContentPage.ToolbarItems> | |
<ContentPage.Content> | |
<StackLayout> | |
<Entry Placeholder="Team Name/Title" | |
Text="{Binding Title}" | |
Margin="4" /> | |
<Entry Placeholder="Manager name" | |
Text="{Binding Manager}" | |
Margin="4" /> | |
<Entry Placeholder="City name" | |
Text="{Binding City}" | |
Margin="4" /> | |
<Entry Placeholder="Name of the stadium" | |
Text="{Binding StadiumName}" | |
Margin="4" /> | |
</StackLayout> | |
</ContentPage.Content> | |
</ContentPage> |
Nothing new 😃
Now create new EditTeamViewModel, and put this code inside of it:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using System.Windows.Input; | |
using Xamarin.Forms; | |
using Xamarin.Forms_Realm.Models; | |
namespace Xamarin.Forms_Realm.ViewModels { | |
class EditTeamViewModel : BaseViewModel { | |
private string title; | |
public string Title { | |
get { return title; } | |
set { | |
title = value; | |
OnPropertyChanged("Title"); | |
} | |
} | |
private string manager; | |
public string Manager { | |
get { return manager; } | |
set { | |
manager = value; | |
OnPropertyChanged("Manager"); | |
} | |
} | |
private string city; | |
public string City { | |
get { return city; } | |
set { | |
city = value; | |
OnPropertyChanged("City"); | |
} | |
} | |
private string stadiumName; | |
public string StadiumName { | |
get { return stadiumName; } | |
set { | |
stadiumName = value; | |
OnPropertyChanged("StadiumName"); | |
} | |
} | |
public ICommand SaveTeamCommand { get; private set; } | |
private string _teamId; | |
public EditTeamViewModel(string teamId) { | |
Realm context = Realm.GetInstance(); | |
var team = context.Find<Team>(teamId); | |
_teamId = team.TeamId; | |
Title = team.Title; | |
Manager = team.Manager; | |
StadiumName = team.StadiumName; | |
City = team.City; | |
SaveTeamCommand = new Command(SaveTeam); | |
} | |
async void SaveTeam() { | |
Realm context = Realm.GetInstance(); | |
var team = context.Find<Team>(_teamId); | |
context.Write(() => { | |
team.Title = Title; | |
team.Manager = Manager; | |
team.StadiumName = StadiumName; | |
team.City = City; | |
context.Add<Team>(team, update: true); | |
}); | |
await Application.Current.MainPage.Navigation.PopAsync(); | |
} | |
} | |
} |
But in the other hand SaveTeam method is very interesting. SaveTeam method will be called when user click on the "Save" toolbar item.
In the SaveTeam we are getting the object by Id... and interesting part is to open that Write method and inside of the delegate we are setting the new values of that team object. If you try to set it outside of the Write method and delegate inside of it you will get the exception: Attempting to modify object outside of a write transaction.
So it is very important to keep in mind that all update operations you need to do inside of Write.
When we are done with setting new values, all we need to do is to commit that changes, in Realm you can update the object in database using Add method, but as second parameter "update" which is of the type bool, it is self explanatory what to do here, so just set it to true and your object will be updated.
Let's test this 😊
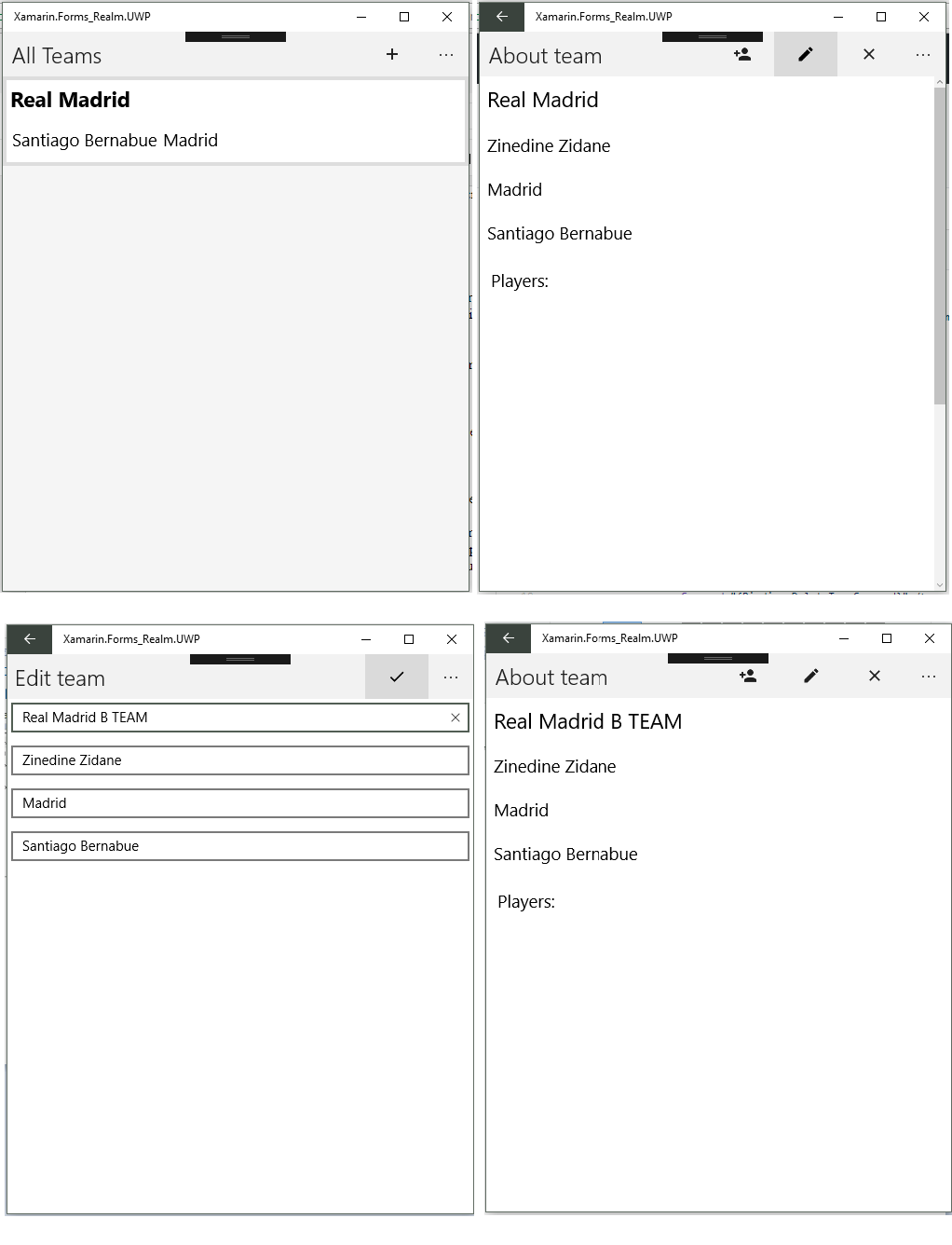
Looks good to me.
With this example we have our CRUD with Realm mobile database completed for Team class/table.
In the next steps I will cover how to add new and update existing one player with foreign object to the Team, remember our one to many relationship for these two classes. You can try this by yourself, following this tutorial you have all knowledge to do that.
Add new player to the team:
Inside of the team details page we have one toolbar item for adding new players, so open your TeamDetailsViewModel and set command to open AddPlayerPage and pass teamId to id, in my gists above I have already done that.If you are not, create new AddPlayerPage and XAML part should looks like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8" ?> | |
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms" | |
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" | |
x:Class="Xamarin.Forms_Realm.Views.AddPlayerPage" | |
Title="Add new player"> | |
<ContentPage.ToolbarItems> | |
<ToolbarItem Text="Save" | |
Icon="ic_save.png" | |
Command="{Binding SavePlayerCommand}" /> | |
</ContentPage.ToolbarItems> | |
<ContentPage.Content> | |
<StackLayout> | |
<Label Text="{Binding TeamName, StringFormat='New player for: {0}'}" | |
Margin="4,12,4,4" /> | |
<Entry Placeholder="Full name" | |
Text="{Binding Name}" | |
Margin="4" /> | |
<Entry Placeholder="Position" | |
Text="{Binding Position}" | |
Margin="4" /> | |
<Entry Placeholder="Jersey Number" | |
Text="{Binding JerseyNumber}" | |
Margin="4" /> | |
</StackLayout> | |
</ContentPage.Content> | |
</ContentPage> |
code behind for this XAML page looks like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using Xamarin.Forms; | |
using Xamarin.Forms.Xaml; | |
using Xamarin.Forms_Realm.Models; | |
using Xamarin.Forms_Realm.ViewModels; | |
namespace Xamarin.Forms_Realm.Views { | |
[XamlCompilation(XamlCompilationOptions.Compile)] | |
public partial class AddPlayerPage : ContentPage { | |
private string _teamId; | |
public AddPlayerPage(string teamId) { | |
InitializeComponent(); | |
_teamId = teamId; | |
} | |
protected override void OnAppearing() { | |
BindingContext = new AddPlayerViewModel(_teamId); | |
} | |
} | |
} |
As you can see from TeamDetailsPage we are passing the teamId value to the AddPlayerPage and when we are setting the BindingContext we are passing the teamId to the AddPlayerViewModel:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using System.Windows.Input; | |
using Xamarin.Forms; | |
using Xamarin.Forms_Realm.Models; | |
namespace Xamarin.Forms_Realm.ViewModels { | |
public class AddPlayerViewModel : BaseViewModel { | |
private string teamName; | |
public string TeamName { | |
get { return teamName; } | |
set { | |
teamName = value; | |
OnPropertyChanged("TeamName"); | |
} | |
} | |
private string name; | |
public string Name { | |
get { return name; } | |
set { | |
name = value; | |
OnPropertyChanged("Name"); | |
} | |
} | |
private string position; | |
public string Position { | |
get { return position; } | |
set { | |
position = value; | |
OnPropertyChanged("Position"); | |
} | |
} | |
private string jerseyNumber; | |
public string JerseyNumber { | |
get { return jerseyNumber; } | |
set { | |
jerseyNumber = value; | |
OnPropertyChanged("JerseyNumber"); | |
} | |
} | |
public ICommand SavePlayerCommand { get; private set; } | |
private string _teamId; | |
private Realm context; | |
public AddPlayerViewModel(string teamId) { | |
_teamId = teamId; | |
context = Realm.GetInstance(); | |
var team = context.All<Team>().Where(t => t.TeamId == teamId).FirstOrDefault(); | |
TeamName = team.Title; | |
SavePlayerCommand = new Command(SavePlayer); | |
} | |
void SavePlayer() { | |
var team = context.All<Team>().Where(t => t.TeamId == _teamId).FirstOrDefault(); | |
context.Write(() => { | |
context.Add<Player>(new Player() { | |
JerseyNumber = Int32.Parse(JerseyNumber), | |
Name = Name, | |
Position = Position, | |
Team = team | |
}); | |
}); | |
Application.Current.MainPage.Navigation.PopAsync(); | |
} | |
} | |
} |
In the constructor we are creating real object called context in the standard way that we learned from past steps in this tutorial.
The main part is in SavePlayer method.
First step is to fetch team by id, for that team we will add new player. As you know SaveMethod is called when toolbar item is clicked.
At the line 71 we are calling the write method and with delegate in it we are adding new player and for foreign property Team we are setting the team that we have from earlier steps.
Now run the app and test it:
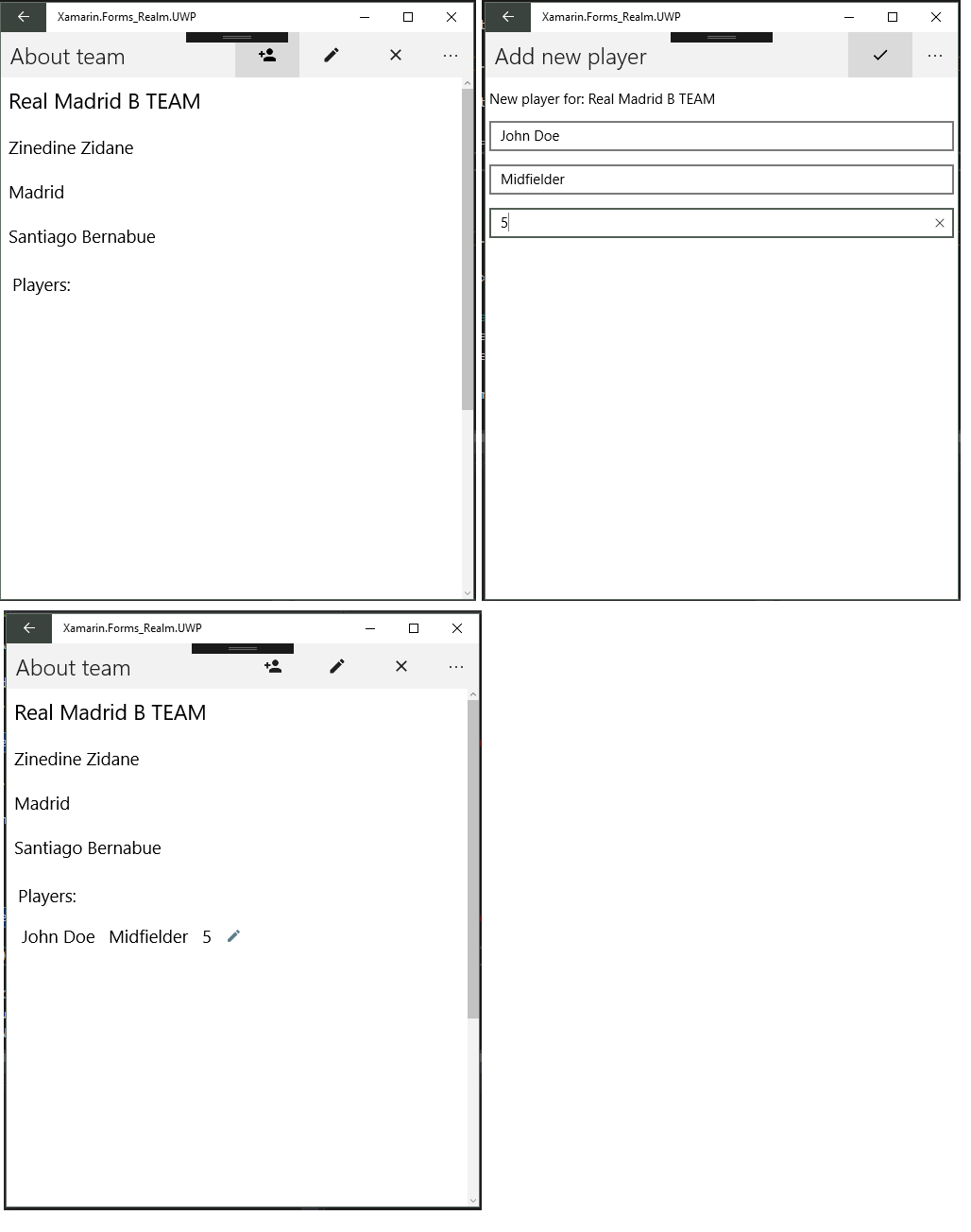
It works!
One last thing is to do update/edit page for Player.
As you can see in our TeamDetaisl.xaml.cs we have one event called OnPlayerTapped where we are opening the EditPlayerPage and we are passing the playerId to it.
EditPlayerPage is very similar to the Add page and it looks like this:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8" ?> | |
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms" | |
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" | |
x:Class="Xamarin.Forms_Realm.Views.EditPlayerPage" | |
Title="Edit player"> | |
<ContentPage.ToolbarItems> | |
<ToolbarItem Text="Save" | |
Icon="ic_save.png" | |
Command="{Binding SavePlayerCommand}" /> | |
</ContentPage.ToolbarItems> | |
<ContentPage.Content> | |
<StackLayout> | |
<Label Text="{Binding TeamName, StringFormat='Playing for: {0}'}" | |
Margin="4,12,4,4" /> | |
<Entry Placeholder="Full name" | |
Text="{Binding Name}" | |
Margin="4" /> | |
<Entry Placeholder="Position" | |
Text="{Binding Position}" | |
Margin="4" /> | |
<Entry Placeholder="Jersey Number" | |
Text="{Binding JerseyNumber}" | |
Margin="4" /> | |
</StackLayout> | |
</ContentPage.Content> | |
</ContentPage> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using Xamarin.Forms; | |
using Xamarin.Forms.Xaml; | |
using Xamarin.Forms_Realm.ViewModels; | |
namespace Xamarin.Forms_Realm.Views { | |
[XamlCompilation(XamlCompilationOptions.Compile)] | |
public partial class EditPlayerPage : ContentPage { | |
private string _playerId; | |
public EditPlayerPage(string playerId) { | |
InitializeComponent(); | |
_playerId = playerId; | |
} | |
protected override void OnAppearing() { | |
BindingContext = new EditPlayerViewModel(_playerId); | |
} | |
} | |
} |
... and our view-model is here:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using Realms; | |
using System; | |
using System.Collections.Generic; | |
using System.Linq; | |
using System.Text; | |
using System.Threading.Tasks; | |
using System.Windows.Input; | |
using Xamarin.Forms; | |
using Xamarin.Forms_Realm.Models; | |
namespace Xamarin.Forms_Realm.ViewModels { | |
public class EditPlayerViewModel : BaseViewModel { | |
private string teamName; | |
public string TeamName { | |
get { return teamName; } | |
set { | |
teamName = value; | |
OnPropertyChanged("TeamName"); | |
} | |
} | |
private string name; | |
public string Name { | |
get { return name; } | |
set { | |
name = value; | |
OnPropertyChanged("Name"); | |
} | |
} | |
private string position; | |
public string Position { | |
get { return position; } | |
set { | |
position = value; | |
OnPropertyChanged("Position"); | |
} | |
} | |
private string jerseyNumber; | |
public string JerseyNumber { | |
get { return jerseyNumber; } | |
set { | |
jerseyNumber = value; | |
OnPropertyChanged("JerseyNumber"); | |
} | |
} | |
public ICommand SavePlayerCommand { get; private set; } | |
private string _playerId; | |
public EditPlayerViewModel(string playerId) { | |
Realm context = Realm.GetInstance(); | |
var player = context.Find<Player>(playerId); | |
_playerId = player.PlayerId; | |
TeamName = player.Team.Title; | |
Name = player.Name; | |
Position = player.Position; | |
JerseyNumber = player.JerseyNumber.ToString(); | |
SavePlayerCommand = new Command(SavePlayer); | |
} | |
void SavePlayer() { | |
Realm context = Realm.GetInstance(); | |
var player = context.Find<Player>(_playerId); | |
context.Write(() => { | |
player.Position = Position; | |
player.Name = Name; | |
player.JerseyNumber = Int32.Parse(JerseyNumber); | |
context.Add<Player>(player, update: true); | |
}); | |
Application.Current.MainPage.Navigation.PopAsync(); | |
} | |
} | |
} |
I hope this tutorial was helpful for you. My goal with this tutorial was to show CRUD examples using this great Realm mobile database. I am sure that I will use it in the future. If anything was blurry you can write me in the comment section down bellow. If this was helpful you can also write it, and share it with your friends.
Best regards! Almir Vuk
8 comments
Hi Almir,
ReplyDeleteFirst thanks for sharing that concise tutorial. How about Offl i ne Sync capability ? Are the objects persisted when the apo is closed and restarted? Could you provide some sample to use a REST API (classic Web Api Controller or Azure Mobile Apps Custom controller)?
Best
Eric
Hi Erich,
DeleteThank you for the comment. I am glad that tutorial was helpful.
If we are talking about built in sync there is something called "Realm mobile platform" you can see here more about it: https://realm.io/products/realm-mobile-platform/
In this tutorial I am covering only the database part, as replacement for the SQLite... I am not sure what you mean by this "Are the objects persisted when the apo is closed and restarted?" if you can explain it in the another comment, yes data is in your app on device, after closing and opening again just like normal database (if you are asking that).
And about more tutorials, yes I will make some of them in the future, right now I am working on my final school project and I'm less active on blog, but I promise more tutorials with realm.
Best regards!
Thank you for your great work!
ReplyDeleteCan I translate this article into Japanese and post it on Qiita(service that shares programming knowledge with programmers)? Of course I will specify the source(the title of this article and the url).
A lot of engineers of the JXUG(Japan Xamarin User Group) are interested in this technology and your article, but there is little information written in Japanese on Xamarin with Realm.
So please let me introduce to your wonderful tutorial to Japanese!
Yes you can, but under one condition and that is to sign me as an author of the blog post and put original (this) blog post URL.
DeleteBest regards!
Excellent tutorial thanks for your apotes to knowledge, in the next few days I will give a conference on local data in xamarin.forms and I will reorganize the conference to talk about this topic and make an alucion to your blog with this link, Thank you very much for Your contribution, after the conference, I'll tell you how I was, cordially @ josetobenito
ReplyDeleteHi Jose,
DeleteI am very glad that my tutorial was helpful for you, good luck at conference!
Best regards!
Toolbar items not displaying.
ReplyDeleteHi,
DeleteHave you added the icons to your platform specific projects?